mirror of
https://gitlab.easter-eggs.com/ee/ldapsaisie.git
synced 2024-07-05 17:37:46 +02:00
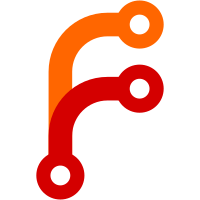
- Ajout d'un LSformElement Date : -> includes/class/class.LSattr_ldap_date.php -> includes/class/class.LSattr_html_date.php -> includes/class/class.LSformElement_date.php -> includes/class/class.LSformRule_date.php -> includes/libs/jscalendar -> includes/js/LSformElement_date.js -> includes/js/LSformElement_date_field.js -> templates/images/calendar.png - LSformElement : Ajout d'une méthode exportValues() utilisée par LSform::exportValues() - LSform : -> Utlisation de LSformElement::exportValues() pour exporter les données du formulaire -> Méthode setValuesFromPostData() est désormais invoqué à chaque invocation de la méthode validate() - LSformElement_select_object : Ajout d'une méthode exportValues() pour coller au nouveau mode d'exportation des données de l'annuaire - LSldapObjet : Correction d'un bug potentiel (foreach sur une variable à false) dans la méthode updateData() - LSsession : -> Méthode addCssFile() & addJSscript() : ajout d'un paramètre pour la possibilité d'inclusion de fichier externe (hors des dossiers par défaut ex: les libs) -> Utilisation de la Constante LS_CSS_DIR au lieu d'une chemin en dure -> Paramètrage JS depuis Php : -> Méthode addJSconfigParam() : ajouter un paramètre de config. JS -> Méthode displayTemplate() adaptée pour -> top.tpl : adapté pour afficher une div contenant les paramètres JSONisés -> LSdefault.css : adapté pour ne pas afficher la div contenant les params. -> LSdefault.js : récupère les informations et Initialise une variable javascript LSjsConfig - LSconfirmBox : Correction d'un debug : "delete this;"
119 lines
4.4 KiB
PHP
119 lines
4.4 KiB
PHP
<?php
|
|
|
|
/**
|
|
* File: calendar.php | (c) dynarch.com 2004
|
|
* Distributed as part of "The Coolest DHTML Calendar"
|
|
* under the same terms.
|
|
* -----------------------------------------------------------------
|
|
* This file implements a simple PHP wrapper for the calendar. It
|
|
* allows you to easily include all the calendar files and setup the
|
|
* calendar by instantiating and calling a PHP object.
|
|
*/
|
|
|
|
define('NEWLINE', "\n");
|
|
|
|
class DHTML_Calendar {
|
|
var $calendar_lib_path;
|
|
|
|
var $calendar_file;
|
|
var $calendar_lang_file;
|
|
var $calendar_setup_file;
|
|
var $calendar_theme_file;
|
|
var $calendar_options;
|
|
|
|
function DHTML_Calendar($calendar_lib_path = '/calendar/',
|
|
$lang = 'en',
|
|
$theme = 'calendar-win2k-1',
|
|
$stripped = true) {
|
|
if ($stripped) {
|
|
$this->calendar_file = 'calendar_stripped.js';
|
|
$this->calendar_setup_file = 'calendar-setup_stripped.js';
|
|
} else {
|
|
$this->calendar_file = 'calendar.js';
|
|
$this->calendar_setup_file = 'calendar-setup.js';
|
|
}
|
|
$this->calendar_lang_file = 'lang/calendar-' . $lang . '.js';
|
|
$this->calendar_theme_file = $theme.'.css';
|
|
$this->calendar_lib_path = preg_replace('/\/+$/', '/', $calendar_lib_path);
|
|
$this->calendar_options = array('ifFormat' => '%Y/%m/%d',
|
|
'daFormat' => '%Y/%m/%d');
|
|
}
|
|
|
|
function set_option($name, $value) {
|
|
$this->calendar_options[$name] = $value;
|
|
}
|
|
|
|
function load_files() {
|
|
echo $this->get_load_files_code();
|
|
}
|
|
|
|
function get_load_files_code() {
|
|
$code = ( '<link rel="stylesheet" type="text/css" media="all" href="' .
|
|
$this->calendar_lib_path . $this->calendar_theme_file .
|
|
'" />' . NEWLINE );
|
|
$code .= ( '<script type="text/javascript" src="' .
|
|
$this->calendar_lib_path . $this->calendar_file .
|
|
'"></script>' . NEWLINE );
|
|
$code .= ( '<script type="text/javascript" src="' .
|
|
$this->calendar_lib_path . $this->calendar_lang_file .
|
|
'"></script>' . NEWLINE );
|
|
$code .= ( '<script type="text/javascript" src="' .
|
|
$this->calendar_lib_path . $this->calendar_setup_file .
|
|
'"></script>' );
|
|
return $code;
|
|
}
|
|
|
|
function _make_calendar($other_options = array()) {
|
|
$js_options = $this->_make_js_hash(array_merge($this->calendar_options, $other_options));
|
|
$code = ( '<script type="text/javascript">Calendar.setup({' .
|
|
$js_options .
|
|
'});</script>' );
|
|
return $code;
|
|
}
|
|
|
|
function make_input_field($cal_options = array(), $field_attributes = array()) {
|
|
$id = $this->_gen_id();
|
|
$attrstr = $this->_make_html_attr(array_merge($field_attributes,
|
|
array('id' => $this->_field_id($id),
|
|
'type' => 'text')));
|
|
echo '<input ' . $attrstr .'/>';
|
|
echo '<a href="#" id="'. $this->_trigger_id($id) . '">' .
|
|
'<img align="middle" border="0" src="' . $this->calendar_lib_path . 'img.gif" alt="" /></a>';
|
|
|
|
$options = array_merge($cal_options,
|
|
array('inputField' => $this->_field_id($id),
|
|
'button' => $this->_trigger_id($id)));
|
|
echo $this->_make_calendar($options);
|
|
}
|
|
|
|
/// PRIVATE SECTION
|
|
|
|
function _field_id($id) { return 'f-calendar-field-' . $id; }
|
|
function _trigger_id($id) { return 'f-calendar-trigger-' . $id; }
|
|
function _gen_id() { static $id = 0; return ++$id; }
|
|
|
|
function _make_js_hash($array) {
|
|
$jstr = '';
|
|
reset($array);
|
|
while (list($key, $val) = each($array)) {
|
|
if (is_bool($val))
|
|
$val = $val ? 'true' : 'false';
|
|
else if (!is_numeric($val))
|
|
$val = '"'.$val.'"';
|
|
if ($jstr) $jstr .= ',';
|
|
$jstr .= '"' . $key . '":' . $val;
|
|
}
|
|
return $jstr;
|
|
}
|
|
|
|
function _make_html_attr($array) {
|
|
$attrstr = '';
|
|
reset($array);
|
|
while (list($key, $val) = each($array)) {
|
|
$attrstr .= $key . '="' . $val . '" ';
|
|
}
|
|
return $attrstr;
|
|
}
|
|
};
|
|
|
|
?>
|